2015-07-22 12:55:44 +02:00
|
|
|
# servant-js
|
2014-12-08 12:01:56 +01:00
|
|
|
|
|
|
|
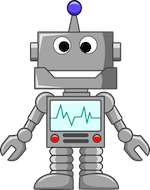
|
|
|
|
|
2015-11-02 19:55:13 +01:00
|
|
|
This library lets you derive automatically Javascript functions that let you query each endpoint of a *servant* webservice.
|
|
|
|
|
|
|
|
It contains a powerful system allowing you to generate functions for several frameworks (Angular, AXios, JQuery) as well as
|
|
|
|
vanilla (framework-free) javascript code.
|
2014-12-08 12:01:56 +01:00
|
|
|
|
|
|
|
## Example
|
|
|
|
|
2015-12-01 06:38:16 +01:00
|
|
|
Read more about the following example [here](https://github.com/haskell-servant/servant/tree/master/servant-js/examples#examples).
|
2014-12-08 12:01:56 +01:00
|
|
|
|
|
|
|
``` haskell
|
|
|
|
{-# LANGUAGE DataKinds #-}
|
|
|
|
{-# LANGUAGE TypeOperators #-}
|
|
|
|
{-# LANGUAGE DeriveGeneric #-}
|
|
|
|
{-# LANGUAGE GeneralizedNewtypeDeriving #-}
|
|
|
|
|
|
|
|
import Control.Concurrent.STM
|
|
|
|
import Control.Monad.IO.Class
|
|
|
|
import Data.Aeson
|
|
|
|
import Data.Proxy
|
|
|
|
import GHC.Generics
|
|
|
|
import Network.Wai.Handler.Warp (run)
|
|
|
|
import Servant
|
2015-11-02 19:55:13 +01:00
|
|
|
import Servant.JS
|
2014-12-08 12:01:56 +01:00
|
|
|
import System.FilePath
|
|
|
|
|
|
|
|
-- * A simple Counter data type
|
|
|
|
newtype Counter = Counter { value :: Int }
|
|
|
|
deriving (Generic, Show, Num)
|
|
|
|
|
|
|
|
instance ToJSON Counter
|
|
|
|
|
|
|
|
-- * Shared counter operations
|
|
|
|
|
|
|
|
-- Creating a counter that starts from 0
|
|
|
|
newCounter :: IO (TVar Counter)
|
|
|
|
newCounter = newTVarIO 0
|
|
|
|
|
|
|
|
-- Increasing the counter by 1
|
|
|
|
counterPlusOne :: MonadIO m => TVar Counter -> m Counter
|
|
|
|
counterPlusOne counter = liftIO . atomically $ do
|
|
|
|
oldValue <- readTVar counter
|
|
|
|
let newValue = oldValue + 1
|
|
|
|
writeTVar counter newValue
|
|
|
|
return newValue
|
|
|
|
|
|
|
|
currentValue :: MonadIO m => TVar Counter -> m Counter
|
|
|
|
currentValue counter = liftIO $ readTVarIO counter
|
|
|
|
|
|
|
|
-- * Our API type
|
2015-11-02 19:55:13 +01:00
|
|
|
type TestApi = "counter" :> Post '[JSON] Counter -- endpoint for increasing the counter
|
|
|
|
:<|> "counter" :> Get '[JSON] Counter -- endpoint to get the current value
|
|
|
|
|
|
|
|
type TestApi' = TestApi -- The API we want a JS handler for
|
|
|
|
:<|> Raw -- used for serving static files
|
2014-12-08 12:01:56 +01:00
|
|
|
|
2015-11-02 19:55:13 +01:00
|
|
|
-- this proxy only targets the proper endpoints of our API,
|
|
|
|
-- not the static file serving bit
|
2014-12-08 12:01:56 +01:00
|
|
|
testApi :: Proxy TestApi
|
|
|
|
testApi = Proxy
|
|
|
|
|
2015-11-02 19:55:13 +01:00
|
|
|
-- this proxy targets everything
|
|
|
|
testApi' :: Proxy TestApi'
|
|
|
|
testApi' = Proxy
|
|
|
|
|
2014-12-08 12:01:56 +01:00
|
|
|
-- * Server-side handler
|
|
|
|
|
|
|
|
-- where our static files reside
|
|
|
|
www :: FilePath
|
|
|
|
www = "examples/www"
|
|
|
|
|
|
|
|
-- defining handlers
|
|
|
|
server :: TVar Counter -> Server TestApi
|
|
|
|
server counter = counterPlusOne counter -- (+1) on the TVar
|
|
|
|
:<|> currentValue counter -- read the TVar
|
2015-11-02 19:55:13 +01:00
|
|
|
|
|
|
|
server' :: TVar Counter -> Server TestApi'
|
|
|
|
server counter = server counter
|
2014-12-08 12:01:56 +01:00
|
|
|
:<|> serveDirectory www -- serve static files
|
|
|
|
|
|
|
|
runServer :: TVar Counter -- ^ shared variable for the counter
|
|
|
|
-> Int -- ^ port the server should listen on
|
|
|
|
-> IO ()
|
2015-11-02 19:55:13 +01:00
|
|
|
runServer var port = run port (serve testApi' $ server' var)
|
2014-12-08 12:01:56 +01:00
|
|
|
|
|
|
|
main :: IO ()
|
|
|
|
main = do
|
|
|
|
-- write the JS code to www/api.js at startup
|
2015-11-02 19:55:13 +01:00
|
|
|
writeJSForAPI testApi jquery (www </> "api.js")
|
2014-12-08 12:01:56 +01:00
|
|
|
|
|
|
|
-- setup a shared counter
|
|
|
|
cnt <- newCounter
|
|
|
|
|
|
|
|
-- listen to requests on port 8080
|
|
|
|
runServer cnt 8080
|
2015-04-20 15:38:26 +02:00
|
|
|
```
|